Automatically Open an Entity in a New Tab
Opening a record or an entity in a new tab seems pretty straightforward: Ctrl and click – and we are done, right? So why the need to write a whole blog post about it? Let me explain.
A user could solve this task perfectly fine – but what if you needed to achieve the desired outcome automatically? Imagine the following scenario: your Power App or Model-driven application uses logic to copy a record. Some attributes can directly be applied to the new entity, while others need to be manually inserted by the user. You would need the new entity to pop up for the user to complete the missing data without blocking the original record nor obscuring the navigation.
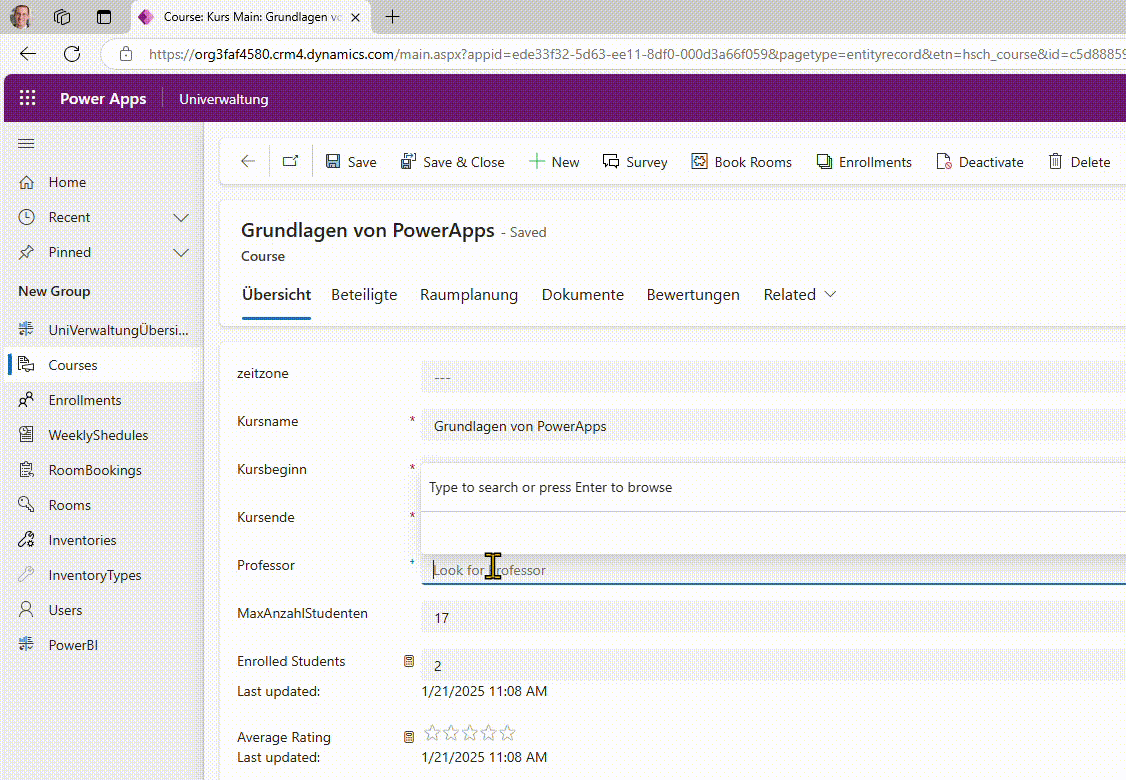
So how do we achieve this? The short answer would be: Use openURL. But let's dive deeper.
The challenge is to get the URL right. Lucky for us, the structure of Dynamics’ URLs is quite clear:
<baseUrl>?appid=<appid>&pagetype=entityrecord&etn=<logicalName>&id=<entityId>[&formid=<formId>]
Looks complicated? Don’t worry. Our task is to simply determine each single part and attach it to form the URL. Let's start.
First, we need the base URL of our current environment. This can be retrieved from the global context.
const baseUrl = Xrm.Utility.getGlobalContext().getClientUrl();
E.g.: https://mybusiness.crm16.dynamics.com/main.aspx
You might consider getting the name of the environment too. With it you can customize the URL depending on the current stage. E.g. mybusiness-dev or mybusiness-test
const environmentName = baseUrl.split("//")?.[1].split(".")?.[0];
You should be familiar with the app Id. But if you like to enhance this feature and open the entity in another app, then you might want to retrieve it from a settings file.
The entity logical name and id depend on your use case and which entity you like to open. But it shouldn’t be a problem for you to get them.
As a bonus, you could also specify the form which should be used. But this is not mandatory. In case you would like to specify it, just add &formid=<formId>
to the end of the URL.
Now that we have all parts, let’s put them together.
const myUrl = `${baseUrl}/main.aspx?appid=${appId}&pagetype=entityrecord&etn=${entityLogicalName}&id=${entityId}&formid=${formId}`;
Hooray. We have a nice URL. The last remaining step is to call it:
Xrm.Navigation.openUrl(myUrl);
In the end it comes down to this little function.
static openInNewTab(entityId: string, entityLogicalName: string) {
const baseUrl = Xrm.Utility.getGlobalContext().getClientUrl();
const caseServiceUrl = `${baseUrl}/main.aspx?appid=<your App Id>&pagetype=entityrecord&etn=${entityLogicalName}&id=${entityId}`;
Xrm.Navigation.openUrl(caseServiceUrl);
}
I hope you find this post useful and will use this functionality in your next project or anytime you would like to open an entity in a new tab or even in another app.